Step 0: Setting Up Your Development Environment
April 30, 2021
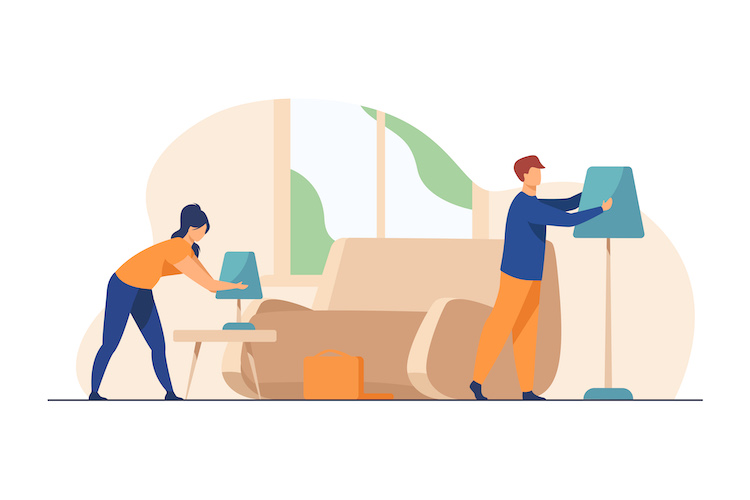
To start coding in JavaScript, we need an environment that can run the JavaScript code that we write. There are different kinds of environments we can use for executing JavaScript code. In this chapter, we will explore some of those options.
Running JavaScript Inside the Developer Console
JavaScript is the programming language used for building web applications. That is why every browser is capable of running JavaScript code. We can even write JavaScript code inside the browser! If we are to launch the Chrome browser and then select View > Developer > JavaScript console
, we can launch the JavaScript console. This interface allows us to write a single line of JavaScript at a time and execute it by pressing the enter key. We can try this by typing console.log(2 + 2);
to the console and pressing enter. We would see the result of this calculation getting displayed. This is a neat feature but is not extremely useful for our purposes since the console only allows for a single line of code to be executed at a time.
All the other prominent browsers (like Safari, Firefox) also have a similar developer console that can run JavaScript code.
Running JavaScript as Part of an HTML File
HTML is a formal language that describes how a document is structured. We can use HTML to describe the content of a web page. We can also use HTML files to load JavaScript files.
<h1>Hello World</h1>
<p>I am learning <strong>JavaScript</strong>!</p>
Here we have a code snippet written in the HTML language. HTML language consists of tags used to describe the elements of a document. Here we have an h1
tag that represents the main title in a document. Then we have a p
tag that describes a paragraph. Inside the p
tag, we have a strong
tag that indicates that the content it wraps is important. These tags help the web browser make sense of the document that we are providing.
HTML code is written inside HTML files. HTML files can also load JavaScript files to be executed by the browser.
Let's first create an HTML file to be loaded by the web browser.
<!DOCTYPE html>
<html>
<body>
<h1>Hello World</h1>
<p>I am learning <strong>JavaScript</strong>!</p>
</body>
</html>
We have added more tags into this HTML code to make it a valid HTML document. We can now drag this file into a web browser or type the full file path into the address bar. The browser will open and render this file.
We can use this HTML file to load JavaScript code as well. Let's create a new file in the same directory as this HTML code with the name main.js
. We can add these two lines of code inside the main.js
file.
console.log("Hello World");
console.log(2 + 2);
Then, we will need to update our HTML file to load this JavaScript file.
<!DOCTYPE html>
<html>
<body>
<h1>Hello World</h1>
<p>I am learning <strong>JavaScript</strong>!</p>
<script src="main.js"></script>
</body>
</html>
We have added a new tag called script
. We can use script
tag to link to an external JavaScript file. It has an attribute called src
that can be used to specify the file path of the file that we want to load. In this example, we need to ensure that the JavaScript file is in the same directory as the HTML file.
Let's launch the JavaScript console after loading this file inside the browser. We would see the result of our JavaScript code is getting displayed in the console. This is a pretty reliable way of running JavaScript code on our computer.
Running JavaScript Online
Since any browser can run JavaScript and HTML, many online editors help to do that in a very easy and practical way.
A couple of services that I use and like are codepen.io, jsbin.com, and codesandbox.io. There are many other services, but these three are the ones that I use the most. We can use all of them to write HTML and JS code inside the browser without creating any files on our computer. They are excellent environments for learning how to code and for prototyping ideas.
Codepen
I love Codepen primarily because of the community aspect of it. It has tons of user-submitted content that shows you all the great things you can do using JavaScript, HTML, and CSS. It is both an amazing tool and a resource. If you go to their online code editor, there are sections that you can use to write HTML, JavaScript, and CSS. There is a white screen that will render your HTML. There is also a tab called Console that you can click to see the results of the JavaScript code that you execute.
Jsbin
I use Jsbin very frequently because it is straightforward to get started. You just need to go to the URL, and you are immediately greeted with a coding editor. To prototype my JavaScript ideas, I select JavaScript, Console, and Output tabs in the menu. JavaScript is the field that you can use to write JavaScript code. Console displays the output of your code in real-time. You can use the Clear button to eliminate the errors that can accumulate as you write your code.
Codesandbox
Codesandbox has excellent features, and it is an incredible tool if you want to prototype a relatively complex idea. It has very advanced features that make it extremely capable as an online editor.
Summary
There are several ways that we can start writing JavaScript. These are:
- Writing it directly inside the browser.
- Writing it inside a
.js
file and loading that file through an HTML file. - Using an online editor.
Of all these options, the first one is not very scalable since it only allows for a single line to be written at a time. We can instead write JavaScript files locally and load them in the browser with an HTML file. We can then use the JavaScript console inside the browser to see the code displayed from our JavaScript file.
The last option that we learned about is an online editor. Online editors are incredibly convenient because we don't have to manage any files on our computers. We just visit a web page, and we are good to go. They are great environments for learning and experimentation.
While following this book, you can either use a local JavaScript file or use an online editor. They should both work for our purposes.
Find me on Social Media