Step 1: Basic Data Types and Variables
April 30, 2021
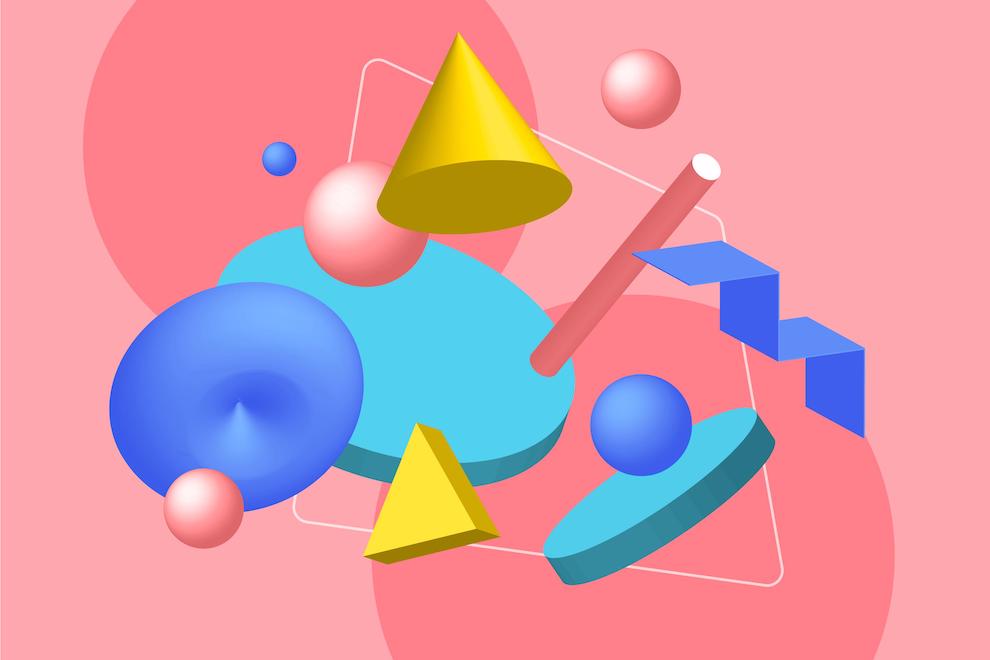
If we enter a number like 42
and execute that code in a JavaScript environment, we would see that it runs without any errors. That is a great sign. It means that we wrote something that the JavaScript language understands. The value that we have entered here is of type number
. There are a couple of different types of values that JavaScript understands. Some of these are:
- numbers
- strings
- and boolean values
In this section, we will learn about numbers and strings.
Numbers
As we have seen before, a JavaScript code that only has a number inside would run without any errors. That is because numbers are a native data type in JavaScript. There are other native data types in JavaScript, such as strings and booleans. We will learn about them soon.
JavaScript programs execute from top to bottom unless we use a structure that would alter the program's flow. This means that we can keep adding different numbers on different lines, and JavaScript would not complain when we execute this code.
42;
1;
2;
3;
It is good that we can write things in JavaScript that doesn't make it complain (or crash and burn), but this is hardly impressive. Let's do something that is slightly more useful, like displaying what we are putting in this file on the screen.
There is a programming structure called a function that packs a bunch of operations together for reusability. We will look into what functions are later on. For now, just know that there is a function called console.log
that we can use to display values on the screen. It works like this:
console.log(42);
We write the name of the function and provide a value to it inside parentheses. The function displays that value to the screen when it runs. This particular code displays the number 42
on the screen.
console.log(1);
console.log(2);
console.log(3);
This program would display the number 1, 2, 3
on the screen on separate lines.
We can also write fractional numbers in JavaScript. Such as:
console.log(3.14);
These numbers are sometimes referred to as floating-point numbers.
Operators
We can use mathematical operators to do calculations using numbers. Here are some of the operators that we can use:
+
-
/
*
Using these operators, we can perform mathematical operations between numbers.
console.log(10 + 20 - 5);
The above operation would display 25
to the screen.
Try to guess what would be the result of the following operation:
console.log(10 - 2 * 2);
If you have guessed 16
, you are making a common mistake. The math operators have different precedence. Multiplication and division happen before addition and subtraction. That's why the result would actually be 6
. We would first have 2
multiplied by 2
, and then that result would get subtracted from 10
.
We can force a specific order of operations by using parentheses (()
). The operation that is in the parentheses would happen before the others.
console.log((10 - 2) * 2);
This would result in 16
since we are forcing 10-2
to happen before multiplication with the usage of parentheses.
Let's take a look at our next data type strings.
Strings
If we wrote a text in the JavaScript console and tried to execute that code, we would likely get an error.
hello;
If we wanted to represent textual data in JavaScript, we need to define it as a string
. A string
is anything that is defined in between quotation marks. We can use single quotes ('
) or double quotes ("
) to define a string. The only restriction is that we need to finish the string with the type of quotation mark we have started with.
"hello";
"42";
Consistency is essential in programming. Even if you are doing something wrong, it would be easy to fix if you have been doing it consistently wrong. That is not to say choosing one kind of quotation mark is wrong over the other, but whatever your choice is, it should stay consistent throughout the codebase.
We might encounter a problem with single quotation mark usage if our text also contains a single quotation mark. If we don't want that quotation mark to be interpreted as the end of the text, we can use a backslash character (\
) in front of it.
'don\'t stop moving';
The backslash allows us to escape the common interpretation of the quotation mark.
We can use the addition math operator with strings as well. We can perform addition in between strings and other data types.
console.log("hello" + " " + "world");
This would display "hello world"
on the screen. Notice, we have an empty string in the middle. The space character still counts as a string and provides the spacing we need in between two words.
We can also add a number and a string together.
console.log("hello" + 2);
JavaScript would convert the number to a string, in this case resulting in hello2
. If we try to perform other operations between strings and numbers (like multiplication), we will get a NaN
result, which means not a number. We would rarely want this value to occur in our program. A NaN
would convert other values into NaN
when used in math operations resulting in erroneous results to accumulate in the program.
Variables
One of the most basic functionalities that we would want from a computer program is to store the results of our operations inside its memory. This is made possible by a programming structure called variables.
A variable is a name that points to a value inside the computer memory. We declare a variable name using one of the variable declaration keywords like var
, let
, const
(more on this later) and assign a value to that variable. Here is an example:
const bigNumber = 999999;
From then on, the variable name is a reference to the value that is assigned to it. The =
symbol assigns the value on the right-hand side to the variable name on the left-hand side. As a result, the below operation would display the number that is assigned to the variable.
console.log(bigNumber);
Variables are incredibly useful and essential since they allow us to reference our programs' values at later stages. Here is an example of that:
const piNumber = 3.14159;
const radius = 5;
const areaOfCircle = radius * piNumber * piNumber;
const diameterOfCircle = 2 * radius;
const circumferenceOfCircle = 2 * piNumber * radius;
console.log(areaOfCircle);
console.log(diameterOfCircle);
console.log(circumferenceOfCircle);
First of all, I am sorry to give you an example that makes such heavy use of math and geometry. Quite honestly, the day-to-day practice of programming can have very little to do with mathematics. It is simply not true that you need to be good at math to become a good programmer. Having said that, examples regarding mathematics are useful when most of what we know about programming has to do with numbers usage.
With that apology out of our way, we can see in this example how the usage of variables helps us when building programs. Since we have saved the values for the pi number and the radius inside variables, we could reference those values by just using their names. Here is what the same program would have looked like if it didn't make use of variables.
const areaOfCircle = 5 * 3.14159 * 3.14159;
const diameterOfCircle = 2 * 5;
const circumferenceOfCircle = 2 * 3.14159 * 5;
console.log(areaOfCircle);
console.log(diameterOfCircle);
console.log(circumferenceOfCircle);
This program looks shorter, but there are a couple of problems with it.
- Code is not DRY
- It uses Magic Numbers
- There is no Single Source of Truth
Let's take a look at what is meant by each of these issues.
Code is not DRY
DRY in software engineering stands for Don't Repeat Yourself and is an essential principle in programming. It means that we should not repetitively do the same things over and over again. Here we are typing the pi number and radius value over and over again across our program. If we needed to change the radius's value, we would have to do it in multiple places. This kind of repetition exposes us to making mistakes. Every time we are typing the pi value, there is a chance that we can make a mistake and mistype it. Following the DRY principle and using a variable to store those values minimizes the risks of such problems.
Usage of Magic Numbers
What do you think the number 5
represents in the above example? If you know geometry well, you might be able to guess that it is the value of the radius of a circle, but that still requires a mental effort. This is what we sometimes refer to as a magic number in programming. It does something and gets us to a result, but it is not entirely clear what it is or how it does that.
It is said that programs are read more than they are written. We should ensure that we create legible programs that are easy to read and do not hide nasty bugs inside. Giving meaningful variable names to values used in our programs ensures that our programs are easy to read and understand.
No Single Source of Truth
In the program above, the value for the radius of the circle is spread throughout the code. There is no single source for that value. If we wanted to change that value, we would need to do it in multiple places. The truth or the knowledge of the value is encoded in multiple places. This can create ambiguity. Imagine there was a mistake in the program, and one line had a different value. How would we know which of the values is the correct one? We can't be sure by looking at the program. Having a single source for that value would give us the confidence that the defined value is the correct one.
We are at the beginning of our programming journey, but these are essential software engineering fundamentals. A structure as simple as variables allows us to alleviate the above problems and write readable and maintainable programs.
Choosing Variable Names
There are some rules and conventions regarding how we choose variable names. Here are some rules that we have to follow:
- Variable names can't start with a number, like
1myVariable
. - Variable names can't contain a space, like
my variable
. - Variable names can't contain a dash character (
-
), likemy-variable
. - Variable names can't make use of reserved words, like
const
.
Of all these rules, the last one might look like it would be the hardest to follow. Reserved words in JavaScript are keywords that are part of the language. For example, the word var
or const
is reserved since it is a JavaScript keyword used to define a variable.
How could we know which variable names are reserved when we are just starting with programming? This is something that you would slowly get accustomed to as you learn more of the language. If we break this rule, our programs will fail to compile with an error that says "SyntaxError: Unexpected token
.
Here are some conventions for choosing variable names. These are not hard rules but helpful and robust suggestions.
Use Camel Casing or Underscores
Use camel casing or underscores (_
) when using a variable name that consists of multiple words to improve legibility.
- Here is an example of a camel-case variable name:
myVariable
. Notice the first letter of the first word is lowercase. Any subsequent words start with an uppercase letter. - Here is an example of the usage of underscore:
my_variable
. In this case, all the words are lowercase but separated by an underscore.
Which of these methods we choose depends on the conventions of the codebase we are working on. In JavaScript, camel-case is generally the convention to go with, but there might be exceptions out there.
Choose Meaningful Names
We should use meaningful variable names to name variables. Imagine the following scenario:
const n = 3.14;
const r = 5;
const a = r * n * n;
console.log(a);
This is a program that calculates the area of a circle with a radius value of 5
. Good luck understanding that by looking at the variable names. We should choose meaningful and explicit variable names when writing our programs. Notice how much better these lines of code read when we name everything more clearly.
const piNumber = 3.14159;
const radius = 5;
const areaOfCircle = radius * piNumber * piNumber;
console.log(areaOfCircle);
Notice that even r
is not a good enough variable name. We need to be explicit enough to make our programs readable for others (and our future self!).
Don't Start With an Uppercase
We shouldn't start variable names with uppercase or so-called title case. We don't do this:
const PiNumber = 3.14;
We might see names that have their first letter uppercase. This is reserved for some special use cases that we will learn about later. Don't use title name variable names until then.
Declaring Variables
So far, we have been declaring our variables using the const
keyword.
const myVariable = 42;
For the longest time, JavaScript only had the var
keyword for the declaration of variables. Later, two other variable declaration keywords, let
and const
got introduced. Here is the difference between each of them.
var Keyword
When we declare a variable name using a var
keyword, that variable name's value can get reassigned. Like this:
var myVariable = 42;
myVariable = 7;
This proved to be problematic because it made it hard to keep track of the variable's value throughout the program's lifetime. That's why we now have the const
keyword to declare variables.
const Keyword
const myVariable = 42;
When we declare a variable using the const
keyword, we can't reassign a different value to that variable. This ensures the value stays consistent throughout the lifetime of our program. It is a limitation, but it is one of those limitations that make our life as programmers easier. 95% of the time, I use the const
keyword to declare my variables. But there are times that we need to reassign the value of the variable. That is when let
keyword comes into play.
let Keyword
let
keyword is very similar to the var
keyword in that it allows for a new value to be assigned to the variable declared using the let
keyword.
let myVariable = 42;
myVariable = 7;
There are some different problems with the usage of the var
keyword that we might not be able to appreciate with the current state of our knowledge. It is primarily regarding the variable's scope, meaning where that variable is going to be accessible from. let
keyword handles that scope issues for us as well. So with the introduction of the let
keyword, I have completely stopped using the var
keyword. const
and let
is going to be the only two keywords that we will be using moving forward. It is still important for us to know that the var
keyword exists, but we would probably see it used less in modern codebases.
Let's take a look at two more things before wrapping up this section.
Semicolons
In JavaScript, we can signal the end of a statement using a semicolon (;
) character. This symbol's usage is not mandatory, but it helps the JavaScript interpreter in some edge cases. Even if we are working with a team that requires us to use semicolons in our code, these kinds of formatting issues are usually handled and automated by code editors nowadays. So semicolon usage is not something that we would have to worry about too much about since it can be automatically handled for us when using a code editor with correct configurations. It is one of those things that is good to know.
Moving forward, I will try to use semicolons in my code examples because I am old school like that.
Comments
There are times that we might not want to have some of the things that we write in our programs to be executed. For example, we might want to leave a comment in our code that explains why we wrote a certain thing the way it is. This can be useful for whoever is going to read the code at a later time. And this might even include ourselves.
This is when comments are useful. It allows us to write things in our program that is not going to be executed by JavaScript.
Whenever we use the //
operator before a line, JavaScript skips executing that line.
// This is a comment
// These lines would not be executed by JavaScript
Summary
We are just starting our programming journey, but we have learned a lot in this chapter. We learned about some of the data types in JavaScript, like numbers and strings. We have also learned about the math operators and the precedence that these operators have. Then, we got introduced to variables. We looked into the rules and conventions that govern the naming of the variables. Additionally, we have learned about let
, const
, and var
keywords used to declare variables. It was important for us to learn the var
keyword, but we will mostly use const
and let
moving forward.
Another thing that was introduced in this chapter is the usage of semicolons at the end of lines. They are not mandatory in JavaScript but can be good to have.
We learned about comments as well. Comments are parts of our programs that are not executed and are generally there for instructional purposes.
Finally, we got introduced to three fundamental programming principles. Importance of DRY, having a Single Source of Truth, and avoiding Magic Numbers. These are very foundational principles in software development and are very useful to keep in mind.
Find me on Social Media